SPI (Serial Peripheral Interface)#
Explore SPI communication protocol and learn how to interface with SPI devices using the DualMCU ONE board. Well walk you through setting up SPI communication and communicating with SPI devices.
SPI Overview#
SPI (Serial Peripheral Interface) is a synchronous, full-duplex, master-slave communication bus. It is commonly used to connect microcontrollers to peripherals such as sensors, displays, and memory devices. The DualMCU ONE development board features SPI communication capabilities, allowing you to interface with a wide range of SPI devices.
Pinout Details#
In the next figure, you can see the SPI pins on the DualMCU ONE board and the corresponding GPIO pins on the ESP32 and RP2040 microcontrollers.
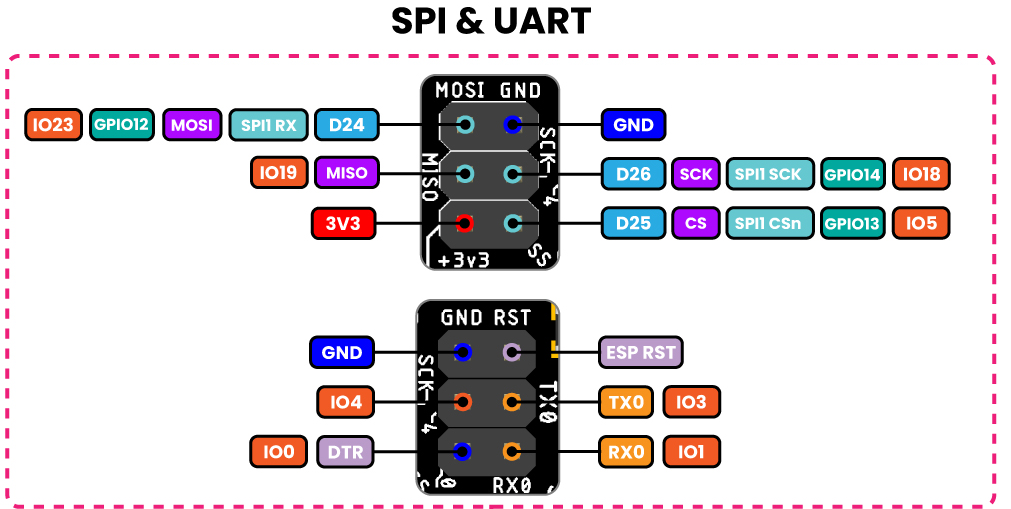
Fig. 29 SPI Pins#
Below is the pinout table for the SPI connections on the DualMCU ONE, detailing the corresponding GPIO connections for both the ESP32 and RP2040 microcontrollers.
PIN |
GPIO ESP32 |
GPIO RP2040 |
---|---|---|
SCK |
18 |
18 |
MISO |
19 |
16 |
MOSI |
23 |
19 |
SS |
5 |
17 |
Caution
ESP32 and RP2040 have conections sharing the same pins, so you recommend to use only one microcontroller at a time.
GPIO ESP32 |
GPIO RP2040 |
---|---|
5 |
13 |
18 |
14 |
23 |
12 |
SPI between ESP32 & RP2040#
DualMCU ONE board was designed to be compatible with both ESP32 and RP2040 microcontrollers. So it is possible to share information between the two microcontrollers using the SPI protocol. For this, we will use the following pins:
Pin |
SPI RP2040 |
Pin |
SPI ESP32 |
---|---|---|---|
SCK |
14 |
SCK |
18 |
MISO |
12 |
MOSI |
23 |
MOSI |
15 |
MISO |
14 |
SS |
13 |
SS |
5 |
READY |
7 |
READY |
33 |
RESET |
16 |
RESET |
RST |
Caution
The connection RST does not exist physically; therefore, it is necessary to establish an external connection.
SDCard SPI#
Warning
Ensure that the Micro SD contain data. We recommend saving multiple files beforehand to facilitate the use.
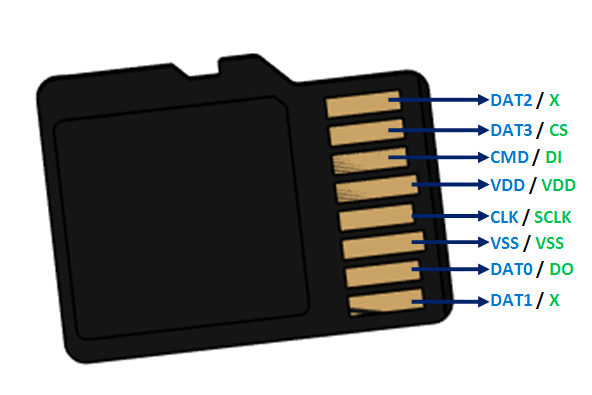
Fig. 30 Micro SD Card Pinout#
Library (MicroPython)#
The dualmcu.py library for MicroPython on ESP32 & RP2040 is compatible with the reader and writer of the Micro SD card. The library provides a simple interface for reading and writing files on the SD card. The library is available on PyPi and can be installed using the Thonny IDE.
Installation
Open Thonny.
Navigate to Tools -> Manage Packages.
Search for
dualmcu
and click Install.
for more information, Check the section
DualMCU ONE Library
Alternatively, download the library from dualmcu.py.
VSPI & HSPI VSPI Interfacing. .. _figura-micro-sd-card-reader:
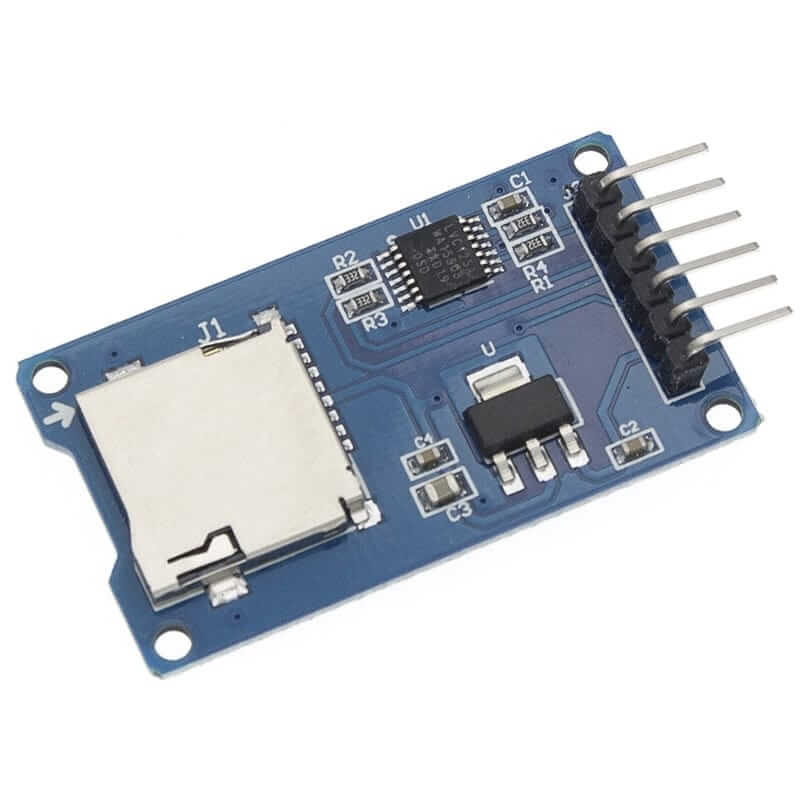
Fig. 31 Micro SD Card external reader#
The conections are as follows:
This table illustrates the connections between the SD card and the GPIO pins on the ESP32 and RP2040 microcontrollers.
SD Card |
Pin Name |
ESP32 |
RP2040 |
---|---|---|---|
D3 |
SS |
5 |
17 |
CMD |
MOSI |
23 |
19 |
VSS |
GND |
||
VDD |
3.3V |
||
CLK |
SCK |
18 |
18 |
D0 |
MISO |
19 |
16 |
Descriptions#
SCK (Serial Clock)
SS (Slave Select)
import machine, os
from dualmcu import *
SCK_PIN = 18
MOSI_PIN = 23
MISO_PIN = 19
CS_PIN = 5
spi = machine.SPI(1, baudrate=100000, polarity=0, phase=0, sck=machine.Pin(SCK_PIN), mosi=machine.Pin(MOSI_PIN), miso=machine.Pin(MISO_PIN))
spi.init()
sd = SDCard(spi, machine.Pin(CS_PIN))
os.mount(sd, '/sd')
os.listdir('/')
print("files ...")
print(os.listdir("/sd"))
HSPI Interfacing.
This table details the connections between the SD card and the ESP32 microcontroller.
SD Card |
ESP32 |
PIN |
---|---|---|
D2 |
12 |
|
D3 |
SS (Slave Select) |
13 |
CMD |
MOSI |
15 |
VSS |
GND |
|
VDD |
3.3V |
|
CLK |
SCK (Serial Clock) |
14 |
VSS |
GND |
|
D0 |
MISO |
2 |
D1 |
4 |
For the test , we will utilize an ESP32 WROM-32E and a SanDisk Micros Ultra card with a capacity of 32 GB.
import machine
import os
from dualmcu import *
# Initialize SPI interface for the SD card
spi = machine.SPI(2, baudrate=1000000, polarity=0, phase=0, sck=machine.Pin(14), mosi=machine.Pin(15), miso=machine.Pin(2))
# Initialize the SD card
sd = SDCard(spi, machine.Pin(13))
# Mount the filesystem
vfs = os.VfsFat(sd)
os.mount(vfs, "/sd")
# List files in the root of the SD card
print("Files in the root of the SD card:")
print(os.listdir("/sd"))
os.umount("/sd")
SPI (Arduino IDE)#
Arduino IDE is compatible for reader and writer of the Micro SD card. The library provides a simple interface for reading and writing files on the SD card.