Exercises with DualMCU - MicroPython
9. Environmental Monitoring System
Objective
Utilize the DTH11 and MQ135 sensors to measure environmental parameters such as humidity, temperature, and air quality in real-time. Visualize the readings in the serial monitor.
NOTE: In this practice, you will use the microcontroller ESP32.
Description
The Environmental Monitoring System is fundamental for measuring and managing parameters such as temperature, humidity, air quality, and other factors for comfortable and secure environments. To continue, we’ll share resources and code to construct an Environmental Monitoring System using the DHT11 and MQ135 sensors with the ESP32 configured with MicroPython.
Materials
Connection Diagram
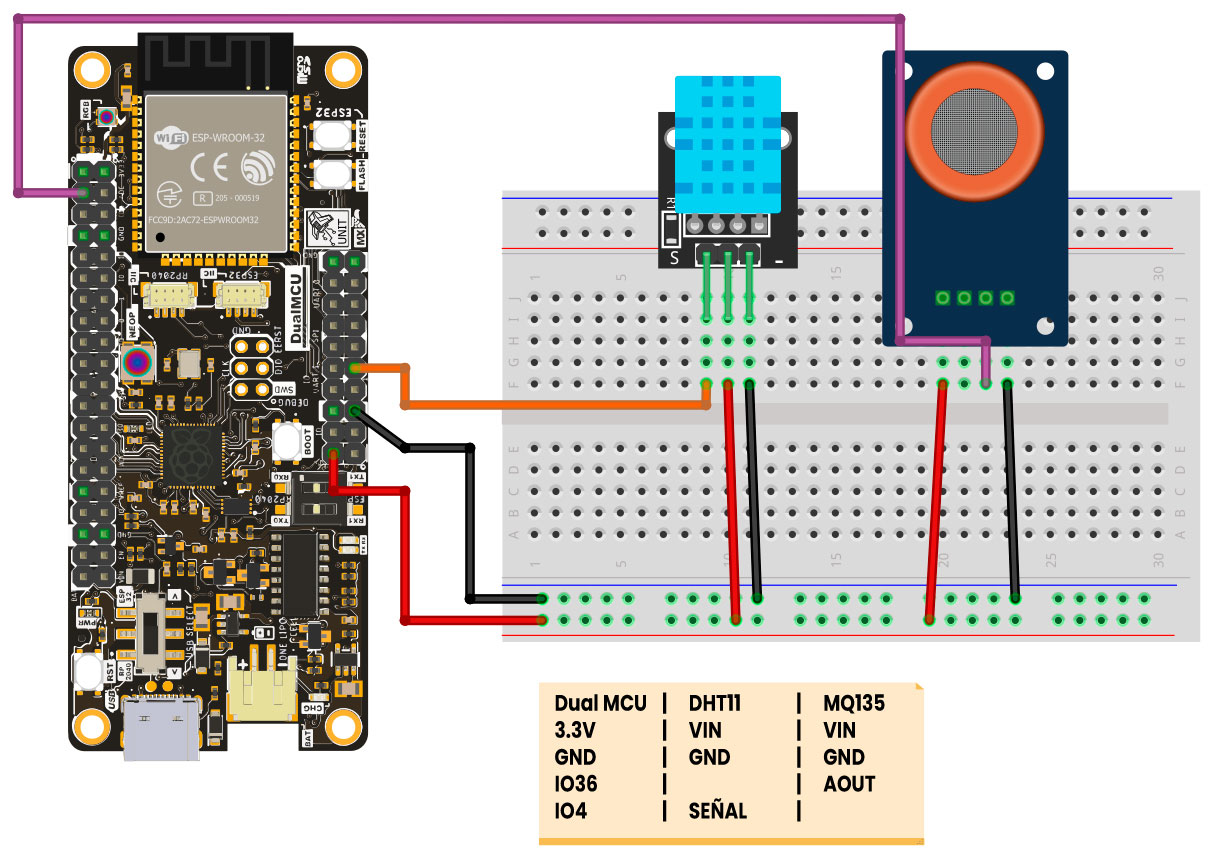
NOTE Remember that when working with the DualMCU, you can switch between microcontrollers using the change switch. For this practice, we will only use the ESP32 microcontroller, so you should switch the change switch to position “B”.
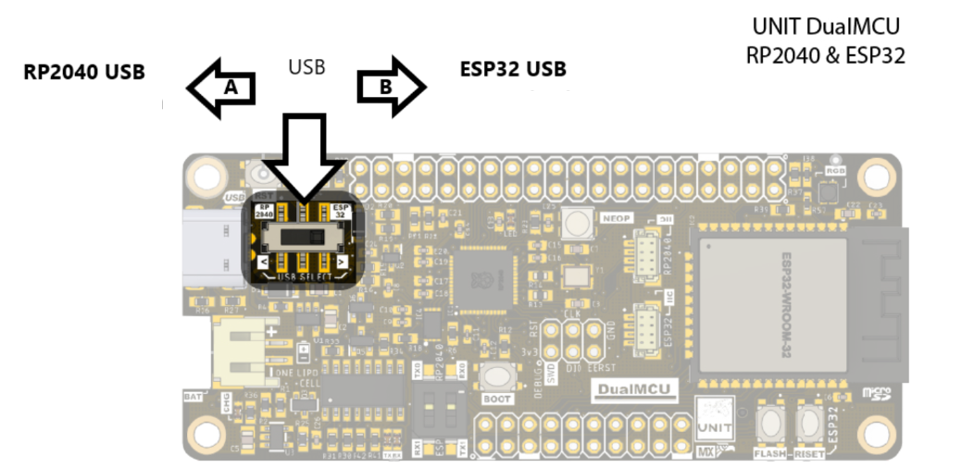
Code
'''
Unit Electronics 2024
(o_
(o_ //\
(/)_ V_/_
version: 0.0.1
revision: 0.0.1
compiler: MicroPython v1.22.0 on 2023-12-27; Generic ESP32 module with ESP32
'''
from dht import DHT11
from machine import Pin, ADC
from time import sleep
sensor = DHT11 (Pin(4))
# configura entrada de adc en el pin 36
adc = ADC(Pin(36))
# configura el rango de lectura de 0 a 3.3v
adc.atten(ADC.ATTN_11DB)
# configura el rango de lectura de 0 a 4095
adc.width(ADC.WIDTH_12BIT)
while True:
try:
sleep(2)
valor = adc.read()
#imprime el valor del adc
print(valor)
sensor.measure()
temp = sensor.temperature()
hum = sensor.humidity()
temp_f = temp * (9/5) + 32.0
print('Temperature: %3.1f C' %temp)
print('Temperature: %3.1f F' %temp_f)
print('Humidity: %3.1f %%' %hum)
except OSError as e:
print('Failed to read sensor.')
Results
This code reads the temperature and humidity from the DHT11 sensor, the air quality from the MQ135 sensor every minute, and prints the read values.
Conclusions
During this practice, knowledge was acquired about the operation of two sensors, which enable the monitoring of temperature, humidity, and air quality. It is imperative to highlight that, in addition to providing the code and the connection diagram, it is necessary to carry out the calibration of the sensors to optimize their utility in a specific application. It is also important to note the feasibility of connecting the ESP32 to a network, thus allowing the sending of the readings acquired to a server or a database.
NOTE: Keep in mind that the presented codes are only examples and may require configuration adjustments according to specific needs and requirements.
Continue with the course OLED Control (I2C)
- License The code presented in this repository is licensed under the GNU General Public License (GPL) version 3.0.
⌨️ with ❤️ from UNIT-Electronics 😊